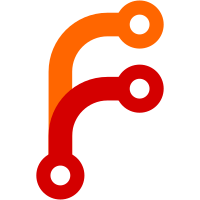
This PR adds readline support to the daemon and monero-wallet-cli. Only GNU readline is supported (e.g. not libedit) and there are cmake checks to ensure this. There is a cmake variable, Readline_ROOT_DIR that can specify a directory to find readline, otherwise some default paths are searched. There is also a cmake option, USE_READLINE, that defaults to ON. If set to ON, if readline is not found, the build continues but without readline support. One negative side effect of using readline is that the color prompt in the wallet-cli now has no color and just uses terminal default. I know how to fix this but it's quite a big change so will tackle another time.
41 lines
640 B
C++
41 lines
640 B
C++
#pragma once
|
|
|
|
#include <streambuf>
|
|
#include <sstream>
|
|
#include <iostream>
|
|
|
|
namespace rdln
|
|
{
|
|
class readline_buffer : public std::stringbuf
|
|
{
|
|
public:
|
|
readline_buffer();
|
|
void start();
|
|
void stop();
|
|
int process();
|
|
bool is_running()
|
|
{
|
|
return m_cout_buf != NULL;
|
|
}
|
|
void get_line(std::string& line);
|
|
void set_prompt(const std::string& prompt);
|
|
|
|
protected:
|
|
virtual int sync();
|
|
|
|
private:
|
|
std::streambuf* m_cout_buf;
|
|
};
|
|
|
|
class suspend_readline
|
|
{
|
|
public:
|
|
suspend_readline();
|
|
~suspend_readline();
|
|
private:
|
|
readline_buffer* m_buffer;
|
|
bool m_restart;
|
|
};
|
|
}
|
|
|