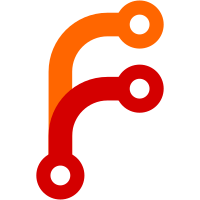
This was the case for monero-wallet-cli already, but not for monerod, which was making it pretty spammy as it was duplicating intended output. Since my original intent was to ensure logs included command output for debugging, this achieves both.
221 lines
7.8 KiB
C++
221 lines
7.8 KiB
C++
// Copyright (c) 2006-2013, Andrey N. Sabelnikov, www.sabelnikov.net
|
|
// All rights reserved.
|
|
//
|
|
// Redistribution and use in source and binary forms, with or without
|
|
// modification, are permitted provided that the following conditions are met:
|
|
// * Redistributions of source code must retain the above copyright
|
|
// notice, this list of conditions and the following disclaimer.
|
|
// * Redistributions in binary form must reproduce the above copyright
|
|
// notice, this list of conditions and the following disclaimer in the
|
|
// documentation and/or other materials provided with the distribution.
|
|
// * Neither the name of the Andrey N. Sabelnikov nor the
|
|
// names of its contributors may be used to endorse or promote products
|
|
// derived from this software without specific prior written permission.
|
|
//
|
|
// THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
|
|
// ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
|
|
// WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
|
|
// DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER BE LIABLE FOR ANY
|
|
// DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
|
|
// (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
|
|
// LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
|
|
// ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
|
|
// (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
|
|
// SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
|
|
//
|
|
|
|
|
|
#ifndef _MISC_LOG_EX_H_
|
|
#define _MISC_LOG_EX_H_
|
|
|
|
#include "static_initializer.h"
|
|
#include "string_tools.h"
|
|
#include "time_helper.h"
|
|
#include "misc_os_dependent.h"
|
|
|
|
#include "syncobj.h"
|
|
|
|
#include <iostream>
|
|
#include <sstream>
|
|
#include <iomanip>
|
|
#include <fstream>
|
|
#include <algorithm>
|
|
#include <list>
|
|
#include <map>
|
|
#include <string>
|
|
#include <time.h>
|
|
#include <boost/cstdint.hpp>
|
|
#include <boost/thread.hpp>
|
|
#include <boost/filesystem.hpp>
|
|
#include <boost/algorithm/string.hpp>
|
|
|
|
#include "easylogging++.h"
|
|
|
|
#define MONERO_DEFAULT_LOG_CATEGORY "default"
|
|
|
|
#define MCFATAL(cat,x) CLOG(FATAL,cat) << x
|
|
#define MCERROR(cat,x) CLOG(ERROR,cat) << x
|
|
#define MCWARNING(cat,x) CLOG(WARNING,cat) << x
|
|
#define MCINFO(cat,x) CLOG(INFO,cat) << x
|
|
#define MCDEBUG(cat,x) CLOG(DEBUG,cat) << x
|
|
#define MCTRACE(cat,x) CLOG(TRACE,cat) << x
|
|
#define MCLOG(level,cat,x) ELPP_WRITE_LOG(el::base::Writer, level, el::base::DispatchAction::NormalLog, cat) << x
|
|
#define MCLOG_FILE(level,cat,x) ELPP_WRITE_LOG(el::base::Writer, level, el::base::DispatchAction::FileOnlyLog, cat) << x
|
|
|
|
#define MCLOG_COLOR(level,cat,color,x) MCLOG(level,cat,"\033[1;" color "m" << x << "\033[0m")
|
|
#define MCLOG_RED(level,cat,x) MCLOG_COLOR(level,cat,"31",x)
|
|
#define MCLOG_GREEN(level,cat,x) MCLOG_COLOR(level,cat,"32",x)
|
|
#define MCLOG_YELLOW(level,cat,x) MCLOG_COLOR(level,cat,"33",x)
|
|
#define MCLOG_BLUE(level,cat,x) MCLOG_COLOR(level,cat,"34",x)
|
|
#define MCLOG_MAGENTA(level,cat,x) MCLOG_COLOR(level,cat,"35",x)
|
|
#define MCLOG_CYAN(level,cat,x) MCLOG_COLOR(level,cat,"36",x)
|
|
|
|
#define MLOG_RED(level,x) MCLOG_RED(level,MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MLOG_GREEN(level,x) MCLOG_GREEN(level,MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MLOG_YELLOW(level,x) MCLOG_YELLOW(level,MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MLOG_BLUE(level,x) MCLOG_BLUE(level,MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MLOG_MAGENTA(level,x) MCLOG_MAGENTA(level,MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MLOG_CYAN(level,x) MCLOG_CYAN(level,MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
|
|
#define MFATAL(x) MCFATAL(MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MERROR(x) MCERROR(MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MWARNING(x) MCWARNING(MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MINFO(x) MCINFO(MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MDEBUG(x) MCDEBUG(MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MTRACE(x) MCTRACE(MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
#define MLOG(level,x) MCLOG(level,MONERO_DEFAULT_LOG_CATEGORY,x)
|
|
|
|
#define MGINFO(x) MCINFO("global",x)
|
|
#define MGINFO_RED(x) MCLOG_RED(el::Level::Info, "global",x)
|
|
#define MGINFO_GREEN(x) MCLOG_GREEN(el::Level::Info, "global",x)
|
|
#define MGINFO_YELLOW(x) MCLOG_YELLOW(el::Level::Info, "global",x)
|
|
#define MGINFO_BLUE(x) MCLOG_BLUE(el::Level::Info, "global",x)
|
|
#define MGINFO_MAGENTA(x) MCLOG_MAGENTA(el::Level::Info, "global",x)
|
|
#define MGINFO_CYAN(x) MCLOG_CYAN(el::Level::Info, "global",x)
|
|
|
|
#define LOG_ERROR(x) MERROR(x)
|
|
#define LOG_PRINT_L0(x) MWARNING(x)
|
|
#define LOG_PRINT_L1(x) MINFO(x)
|
|
#define LOG_PRINT_L2(x) MDEBUG(x)
|
|
#define LOG_PRINT_L3(x) MTRACE(x)
|
|
#define LOG_PRINT_L4(x) MTRACE(x)
|
|
|
|
#define _dbg3(x) MTRACE(x)
|
|
#define _dbg2(x) MDEBUG(x)
|
|
#define _dbg1(x) MDEBUG(x)
|
|
#define _info(x) MINFO(x)
|
|
#define _note(x) MINFO(x)
|
|
#define _fact(x) MINFO(x)
|
|
#define _mark(x) MINFO(x)
|
|
#define _warn(x) MWARNING(x)
|
|
#define _erro(x) MERROR(x)
|
|
|
|
#define MLOG_SET_THREAD_NAME(x) el::Helpers::setThreadName(x)
|
|
|
|
#ifndef LOCAL_ASSERT
|
|
#include <assert.h>
|
|
#if (defined _MSC_VER)
|
|
#define LOCAL_ASSERT(expr) {if(epee::debug::get_set_enable_assert()){_ASSERTE(expr);}}
|
|
#else
|
|
#define LOCAL_ASSERT(expr)
|
|
#endif
|
|
|
|
#endif
|
|
|
|
std::string mlog_get_default_log_path(const char *default_filename);
|
|
void mlog_configure(const std::string &filename_base, bool console);
|
|
void mlog_set_categories(const char *categories);
|
|
void mlog_set_log_level(int level);
|
|
void mlog_set_log(const char *log);
|
|
|
|
namespace epee
|
|
{
|
|
namespace debug
|
|
{
|
|
inline bool get_set_enable_assert(bool set = false, bool v = false)
|
|
{
|
|
static bool e = true;
|
|
if(set)
|
|
e = v;
|
|
return e;
|
|
}
|
|
}
|
|
|
|
|
|
|
|
#define ENDL std::endl
|
|
|
|
#define TRY_ENTRY() try {
|
|
#define CATCH_ENTRY(location, return_val) } \
|
|
catch(const std::exception& ex) \
|
|
{ \
|
|
(void)(ex); \
|
|
LOG_ERROR("Exception at [" << location << "], what=" << ex.what()); \
|
|
return return_val; \
|
|
}\
|
|
catch(...)\
|
|
{\
|
|
LOG_ERROR("Exception at [" << location << "], generic exception \"...\"");\
|
|
return return_val; \
|
|
}
|
|
|
|
#define CATCH_ENTRY_L0(lacation, return_val) CATCH_ENTRY(lacation, return_val)
|
|
#define CATCH_ENTRY_L1(lacation, return_val) CATCH_ENTRY(lacation, return_val)
|
|
#define CATCH_ENTRY_L2(lacation, return_val) CATCH_ENTRY(lacation, return_val)
|
|
#define CATCH_ENTRY_L3(lacation, return_val) CATCH_ENTRY(lacation, return_val)
|
|
#define CATCH_ENTRY_L4(lacation, return_val) CATCH_ENTRY(lacation, return_val)
|
|
|
|
|
|
#define ASSERT_MES_AND_THROW(message) {LOG_ERROR(message); std::stringstream ss; ss << message; throw std::runtime_error(ss.str());}
|
|
#define CHECK_AND_ASSERT_THROW_MES(expr, message) {if(!(expr)) ASSERT_MES_AND_THROW(message);}
|
|
|
|
|
|
#ifndef CHECK_AND_ASSERT
|
|
#define CHECK_AND_ASSERT(expr, fail_ret_val) do{if(!(expr)){LOCAL_ASSERT(expr); return fail_ret_val;};}while(0)
|
|
#endif
|
|
|
|
#ifndef CHECK_AND_ASSERT_MES
|
|
#define CHECK_AND_ASSERT_MES(expr, fail_ret_val, message) do{if(!(expr)) {LOG_ERROR(message); return fail_ret_val;};}while(0)
|
|
#endif
|
|
|
|
#ifndef CHECK_AND_NO_ASSERT_MES_L
|
|
#define CHECK_AND_NO_ASSERT_MES_L(expr, fail_ret_val, l, message) do{if(!(expr)) {LOG_PRINT_L##l(message); /*LOCAL_ASSERT(expr);*/ return fail_ret_val;};}while(0)
|
|
#endif
|
|
|
|
#ifndef CHECK_AND_NO_ASSERT_MES
|
|
#define CHECK_AND_NO_ASSERT_MES(expr, fail_ret_val, message) CHECK_AND_NO_ASSERT_MES_L(expr, fail_ret_val, 0, message)
|
|
#endif
|
|
|
|
#ifndef CHECK_AND_NO_ASSERT_MES_L1
|
|
#define CHECK_AND_NO_ASSERT_MES_L1(expr, fail_ret_val, message) CHECK_AND_NO_ASSERT_MES_L(expr, fail_ret_val, 1, message)
|
|
#endif
|
|
|
|
|
|
#ifndef CHECK_AND_ASSERT_MES_NO_RET
|
|
#define CHECK_AND_ASSERT_MES_NO_RET(expr, message) do{if(!(expr)) {LOG_ERROR(message); return;};}while(0)
|
|
#endif
|
|
|
|
|
|
#ifndef CHECK_AND_ASSERT_MES2
|
|
#define CHECK_AND_ASSERT_MES2(expr, message) do{if(!(expr)) {LOG_ERROR(message); };}while(0)
|
|
#endif
|
|
|
|
enum console_colors
|
|
{
|
|
console_color_default,
|
|
console_color_white,
|
|
console_color_red,
|
|
console_color_green,
|
|
console_color_blue,
|
|
console_color_cyan,
|
|
console_color_magenta,
|
|
console_color_yellow
|
|
};
|
|
|
|
bool is_stdout_a_tty();
|
|
void set_console_color(int color, bool bright);
|
|
void reset_console_color();
|
|
|
|
}
|
|
#endif //_MISC_LOG_EX_H_
|