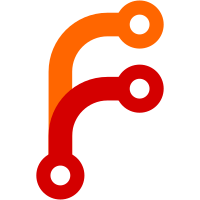
libglim is an Apache-licensed C++ wrapper for lmdb, and rather than rolling our own it seems prudent to use it. Note: lmdb is not included in it, and unless something happens as did with libunbound, should be installed via each OS' package manager or equivalent.
35 lines
1.3 KiB
C++
35 lines
1.3 KiB
C++
#include <functional>
|
|
namespace glim {
|
|
|
|
// http://stackoverflow.com/questions/2121607/any-raii-template-in-boost-or-c0x/
|
|
|
|
/// RAII helper. Keeps the functor and runs it in the destructor.
|
|
/// Example: \code auto unmap = raiiFun ([&]() {munmap (fd, size);}); \endcode
|
|
template<typename Fun> struct RAIIFun {
|
|
Fun _fun;
|
|
RAIIFun (RAIIFun&&) = default;
|
|
RAIIFun (const RAIIFun&) = default;
|
|
template<typename FunArg> RAIIFun (FunArg&& fun): _fun (std::forward<Fun> (fun)) {}
|
|
~RAIIFun() {_fun();}
|
|
};
|
|
|
|
/// The idea to name it `finally` comes from http://www.codeproject.com/Tips/476970/finally-clause-in-Cplusplus.
|
|
/// Example: \code finally unmap ([&]() {munmap (fd, size);}); \endcode
|
|
typedef RAIIFun<std::function<void(void)>> finally;
|
|
|
|
/// Runs the given functor when going out of scope.
|
|
/// Example: \code
|
|
/// auto closeFd = raiiFun ([&]() {close (fd);});
|
|
/// auto unmap = raiiFun ([&]() {munmap (fd, size);});
|
|
/// \endcode
|
|
template<typename Fun> RAIIFun<Fun> raiiFun (const Fun& fun) {return RAIIFun<Fun> (fun);}
|
|
|
|
/// Runs the given functor when going out of scope.
|
|
/// Example: \code
|
|
/// auto closeFd = raiiFun ([&]() {close (fd);});
|
|
/// auto unmap = raiiFun ([&]() {munmap (fd, size);});
|
|
/// \endcode
|
|
template<typename Fun> RAIIFun<Fun> raiiFun (Fun&& fun) {return RAIIFun<Fun> (std::move (fun));}
|
|
|
|
}
|